Supercharge user profiles with ChatGPT and Twilio Segment: unlocking the power of personalization based on sentiment analysis
It's essential to understand your users in order to provide them with personalized and relevant experiences. By leveraging the power of ChatGPT, OpenAI's advanced language model, and Twilio Segment, a leading customer data platform (CDP), you can gain valuable insights from user-generated content and track that data to enrich user profiles. In this recipe, we'll discuss a powerful use case built using ChatGPT to analyze user feedback, and track the data in Segment. We'll also provide a code example in Python with comments to help inspire server-side implementation for your own projects.
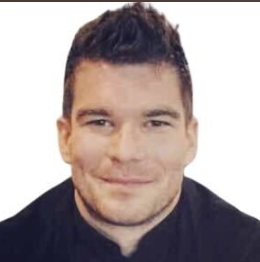
What do you need?
-
ChatGPT access
-
Segment with Track and Identify Calls implemented
Easily personalize customer experiences with first-party data
With a huge integration catalog and plenty of no-code features, Segment provides easy-to-maintain capability to your teams with minimal engineering effort. Great data doesn't have to be hard work!